Simple player. More...
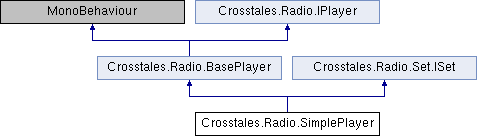
Public Member Functions | |
void | Next () |
Plays the next radio station. More... | |
RadioStation | Next (bool random, RadioFilter _filter=null) |
Plays the next (normal/random) radio station. More... | |
void | Previous () |
Plays the previous radio station. More... | |
RadioStation | Previous (bool random, RadioFilter _filter=null) |
Plays the previous radio station. More... | |
System.Collections.Generic.List< RadioStation > | GetStations (bool random=false, RadioFilter _filter=null) |
Get all RadioStation for a given RadioFilter. More... | |
int | CountStations (RadioFilter _filter=null) |
Count all RadioStation for a given RadioFilter. More... | |
RadioStation | StationFromIndex (bool random=false, int index=-1, RadioFilter _filter=null) |
Radio station from a given index (normal/random) from this set. More... | |
RadioStation | StationFromHashCode (int hashCode) |
Radio station from a hashcode from this set. More... | |
RadioStation | NextStation (bool random=false, RadioFilter _filter=null) |
Next (normal/random) radio station from this set. More... | |
RadioStation | PreviousStation (bool random=false, RadioFilter _filter=null) |
Previous (normal/random) radio station from this set. More... | |
System.Collections.Generic.List< RadioStation > | StationsByName (bool desc=false, RadioFilter _filter=null) |
Returns all radio stations of this set ordered by name. More... | |
System.Collections.Generic.List< RadioStation > | StationsByURL (bool desc=false, RadioFilter _filter=null) |
Returns all radio stations of this set ordered by URL. More... | |
System.Collections.Generic.List< RadioStation > | StationsByFormat (bool desc=false, RadioFilter _filter=null) |
Returns all radio stations of this set ordered by audio format. More... | |
System.Collections.Generic.List< RadioStation > | StationsByStation (bool desc=false, RadioFilter _filter=null) |
Returns all radio stations of this set ordered by station. More... | |
System.Collections.Generic.List< RadioStation > | StationsByBitrate (bool desc=false, RadioFilter _filter=null) |
Returns all radio stations of this set ordered by bitrate. More... | |
System.Collections.Generic.List< RadioStation > | StationsByGenres (bool desc=false, RadioFilter _filter=null) |
Returns all radio stations of this set ordered by genres. More... | |
System.Collections.Generic.List< RadioStation > | StationsByCities (bool desc=false, RadioFilter _filter=null) |
Returns all radio stations of this set ordered by cities. More... | |
System.Collections.Generic.List< RadioStation > | StationsByCountries (bool desc=false, RadioFilter _filter=null) |
Returns all radio stations of this set ordered by countries. More... | |
System.Collections.Generic.List< RadioStation > | StationsByLanguages (bool desc=false, RadioFilter _filter=null) |
Returns all radio stations of this set ordered by languages. More... | |
System.Collections.Generic.List< RadioStation > | StationsByRating (bool desc=false, RadioFilter _filter=null) |
Returns all radio stations of this set ordered by rating. More... | |
void | Load () |
Loads all stations from this set (via providers). More... | |
void | Save (string path, RadioFilter _filter=null) |
Saves all stations from this set as text-file with streams. More... | |
void | RandomizeStations (bool resetIndex=true) |
Randomize all radio stations. More... | |
override void | Play () |
Plays the radio-station. More... | |
override void | Stop () |
Stops the playback of the radio-station. More... | |
override void | Restart (float invokeDelay=Constants.INVOKE_DELAY) |
Restarts the playback of the radio-station. More... | |
override void | Mute () |
Mute the playback of the record. More... | |
override void | UnMute () |
Unmute the playback of the record. More... | |
![]() | |
virtual void | PlayOrStop () |
Plays or stops the radio-station. More... | |
virtual void | MuteOrUnMute () |
Mute or unmute the playback of the record. More... | |
Public Attributes | |
PlaybackStartEvent | OnPlaybackStarted |
PlaybackEndEvent | OnPlaybackEnded |
BufferingStartEvent | OnBufferingStarted |
BufferingEndEvent | OnBufferingEnded |
AudioStartEvent | OnAudioStarted |
AudioEndEvent | OnAudioEnded |
RecordChangeEvent | OnRecordChanged |
StationChangeEvent | OnStationChanged |
FilterChangeEvent | OnFilterChanged |
StationsChangeEvent | OnStationsChanged |
ProviderReadyEvent | OnProviderReadyEvent |
ErrorEvent | OnError |
System.Collections.Generic.List< RadioStation > | Stations => Set != null ? Set.Stations : new System.Collections.Generic.List<RadioStation>() |
System.Collections.Generic.List< RadioStation > | RandomStations => Set != null ? Set.RandomStations : new System.Collections.Generic.List<RadioStation>() |
bool | isReady => Set != null && Set.isReady |
override bool | isPlayback => Player.isPlayback |
override bool | isAudioPlaying => Player.isAudioPlaying |
override bool | isBuffering => Player.isBuffering |
override RecordInfo | RecordInfo => Player.RecordInfo |
override RecordInfo | NextRecordInfo => Player.RecordInfo |
override float | NextRecordDelay => Player.NextRecordDelay |
override long | CurrentBufferSize => Player.CurrentBufferSize |
override long | CurrentDownloadSpeed => Player.CurrentDownloadSpeed |
override int | Channels => Player.Channels |
override int | SampleRate => Player.SampleRate |
Protected Member Functions | |
override void | onAudioStart (RadioStation station) |
override void | onAudioEnd (RadioStation station) |
override void | onAudioPlayTimeUpdate (RadioStation station, float _playtime) |
override void | onErrorInfo (RadioStation station, string info) |
![]() | |
virtual void | onPlaybackStart (RadioStation station) |
virtual void | onPlaybackEnd (RadioStation station) |
virtual void | onBufferingStart (RadioStation station) |
virtual void | onBufferingEnd (RadioStation station) |
virtual void | onBufferingProgressUpdate (RadioStation station, float progress) |
virtual void | onRecordChange (RadioStation station, RecordInfo newRecord) |
virtual void | onRecordPlayTimeUpdate (RadioStation station, RecordInfo record, float playtime) |
virtual void | onNextRecordChange (RadioStation station, RecordInfo nextRecord, float delay) |
virtual void | onNextRecordDelayUpdate (RadioStation station, RecordInfo nextRecord, float delay) |
Protected Attributes | |
override PlaybackStartEvent | onPlaybackStarted => OnPlaybackStarted |
override PlaybackEndEvent | onPlaybackEnded => OnPlaybackEnded |
override BufferingStartEvent | onBufferingStarted => OnBufferingStarted |
override BufferingEndEvent | onBufferingEnded => OnBufferingEnded |
override AudioStartEvent | onAudioStarted => OnAudioStarted |
override AudioEndEvent | onAudioEnded => OnAudioEnded |
override RecordChangeEvent | onRecordChanged => OnRecordChanged |
override ErrorEvent | onError => OnError |
Properties | |
RadioPlayer | Player [get, set] |
'RadioPlayer' from the scene. More... | |
RadioSet | Set [get, set] |
'RadioSet' from the scene. More... | |
RadioFilter | Filter [get, set] |
Global RadioFilter (active if no explicit filter is given). More... | |
bool | RetryOnError [get, set] |
Retry to start the radio on an error. More... | |
int | Retries [get, set] |
Defines how many times should the radio station restart after an error before giving up. More... | |
bool | PlayOnStart [get, set] |
Play a radio on start. More... | |
bool | PlayEndless [get, set] |
Enable endless play. More... | |
bool | PlayRandom [get, set] |
Play the radio stations in random order. More... | |
bool | FollowDirection [get, set] |
In case 'Next' or 'Previous' is called, follow the logical direction through the playlist. More... | |
int? | CurrentStationIndex [get, set] |
int? | CurrentRandomStationIndex [get, set] |
override RadioStation | Station [get, set] |
override bool | HandleFocus [get, set] |
override int | CacheStreamSize [get, set] |
override bool | LegacyMode [get, set] |
override bool | CaptureDataStream [get, set] |
override bool | SkipPreBuffering [get, set] |
override AudioSource | Source [get, protected set] |
override AudioCodec | Codec [get, protected set] |
override float | PlayTime [get, protected set] |
override float | BufferProgress [get, protected set] |
override float | RecordPlayTime [get, protected set] |
override Crosstales.Common.Util.MemoryCacheStream | DataStream [get, protected set] |
override float | Volume [get, set] |
override float | Pitch [get, set] |
override float | StereoPan [get, set] |
override bool | isMuted [get, set] |
![]() | |
static int? | playCounter [get, set] |
static int? | audioCounter [get, set] |
abstract PlaybackStartEvent | onPlaybackStarted [get] |
abstract PlaybackEndEvent | onPlaybackEnded [get] |
abstract BufferingStartEvent | onBufferingStarted [get] |
abstract BufferingEndEvent | onBufferingEnded [get] |
abstract AudioStartEvent | onAudioStarted [get] |
abstract AudioEndEvent | onAudioEnded [get] |
abstract RecordChangeEvent | onRecordChanged [get] |
abstract ErrorEvent | onError [get] |
abstract RadioStation | Station [get, set] |
abstract bool | HandleFocus [get, set] |
abstract int | CacheStreamSize [get, set] |
abstract bool | LegacyMode [get, set] |
abstract bool | CaptureDataStream [get, set] |
abstract bool | SkipPreBuffering [get, set] |
abstract AudioSource | Source [get, protected set] |
abstract AudioCodec | Codec [get, protected set] |
abstract float | PlayTime [get, protected set] |
abstract float | BufferProgress [get, protected set] |
abstract bool | isBuffering [get] |
abstract long | CurrentBufferSize [get] |
abstract bool | isPlayback [get] |
abstract bool | isAudioPlaying [get] |
abstract float | RecordPlayTime [get, protected set] |
abstract RecordInfo | RecordInfo [get] |
abstract RecordInfo | NextRecordInfo [get] |
abstract float | NextRecordDelay [get] |
abstract long | CurrentDownloadSpeed [get] |
abstract Crosstales.Common.Util.MemoryCacheStream | DataStream [get, protected set] |
abstract int | Channels [get] |
abstract int | SampleRate [get] |
abstract float | Volume [get, set] |
abstract float | Pitch [get, set] |
abstract float | StereoPan [get, set] |
abstract bool | isMuted [get, set] |
![]() | |
RadioStation | Station [get, set] |
Current RadioStation of this player. More... | |
bool | HandleFocus [get, set] |
Starts and stops the RadioPlayer depending on the focus and running state. More... | |
int | CacheStreamSize [get, set] |
Size of the cache stream in bytes. More... | |
bool | LegacyMode [get, set] |
Enable or disable legacy mode. Legacy mode disables all record information, but is more stable. More... | |
bool | CaptureDataStream [get, set] |
Capture the encoded PCM-stream from this player. More... | |
bool | SkipPreBuffering [get, set] |
Enable or disable skipping of the pre-buffering. Skip pre-buffering allows for faster playback, but is less resilient. More... | |
AudioSource | Source [get] |
Returns the AudioSource of for this player. More... | |
AudioCodec | Codec [get] |
Returns the codec of for this player. More... | |
float | PlayTime [get] |
Returns the current playtime of this player. More... | |
float | BufferProgress [get] |
Returns the current buffer progress in percent. More... | |
bool | isBuffering [get] |
Is this player buffering? More... | |
long | CurrentBufferSize [get] |
Returns the size of the current buffer in bytes. More... | |
bool | isPlayback [get] |
Is this player in playback-mode? More... | |
bool | isAudioPlaying [get] |
Is this player playing audio? More... | |
float | RecordPlayTime [get] |
Returns the playtime of the current audio record. More... | |
RecordInfo | RecordInfo [get] |
Returns the information about the current audio record. More... | |
RecordInfo | NextRecordInfo [get] |
Returns the information about the next audio record. This information is updated a few seconds before a new record starts. More... | |
float | NextRecordDelay [get] |
Returns the current delay in seconds until the next audio record starts. More... | |
long | CurrentDownloadSpeed [get] |
Returns the current download speed in Bytes per second. More... | |
Crosstales.Common.Util.MemoryCacheStream | DataStream [get] |
Returns the encoded PCM-stream from this player. More... | |
int | Channels [get] |
Current audio channels of the current station. More... | |
int | SampleRate [get] |
Current audio sample rate of the current station. More... | |
float | Volume [get, set] |
Current volume of this player. More... | |
float | Pitch [get, set] |
Current pitch of this player. More... | |
float | StereoPan [get, set] |
Current stereo pan of this player. More... | |
bool | isMuted [get, set] |
Is this player muted? More... | |
![]() | |
System.Collections.Generic.List< RadioStation > | Stations [get] |
List of all loaded RadioStation from all providers. More... | |
System.Collections.Generic.List< RadioStation > | RandomStations [get] |
Returns the list of all randomized RadioStation from this set. More... | |
bool | isReady [get] |
Are all providers of this set ready (= data loaded)? More... | |
int | CurrentStationIndex [get, set] |
Current station index. More... | |
int | CurrentRandomStationIndex [get, set] |
Current random station index. More... | |
Events | |
FilterChange | OnFilterChange |
An event triggered whenever the filter changes. More... | |
StationsChange | OnStationsChange |
An event triggered whenever the stations change. More... | |
ProviderReady | OnProviderReady |
An event triggered whenever all providers are ready. More... | |
StationChange | OnStationChange |
An event triggered whenever an radio station changes. More... | |
![]() | |
PlaybackStart | OnPlaybackStart |
An event triggered whenever the playback starts. More... | |
PlaybackEnd | OnPlaybackEnd |
An event triggered whenever the playback ends. More... | |
BufferingStart | OnBufferingStart |
An event triggered whenever the buffering starts. More... | |
BufferingEnd | OnBufferingEnd |
An event triggered whenever the buffering ends. More... | |
BufferingProgressUpdate | OnBufferingProgressUpdate |
An event triggered whenever the buffering progress changes. More... | |
AudioStart | OnAudioStart |
An event triggered whenever the audio starts. More... | |
AudioEnd | OnAudioEnd |
An event triggered whenever the audio ends. More... | |
AudioPlayTimeUpdate | OnAudioPlayTimeUpdate |
An event triggered whenever the audio playtime changes. More... | |
RecordChange | OnRecordChange |
An event triggered whenever an audio record changes. More... | |
RecordPlayTimeUpdate | OnRecordPlayTimeUpdate |
An event triggered whenever the audio record playtime changes. More... | |
NextRecordChange | OnNextRecordChange |
An event triggered whenever the next record information is available. More... | |
NextRecordDelayUpdate | OnNextRecordDelayUpdate |
An event triggered whenever the next record delay time changes. More... | |
ErrorInfo | OnErrorInfo |
An event triggered whenever an error occurs. More... | |
Additional Inherited Members | |
![]() | |
static bool | isAnyPlayback => playCounter > 0 |
Checks if ANY RadioPlayer is in playback-mode on this system. More... | |
static bool | isAnyAudioPlaying => audioCounter > 0 |
Checks if ANY RadioPlayer playing audio on this system. More... | |
Detailed Description
Simple player.
Member Function Documentation
◆ CountStations()
int Crosstales.Radio.SimplePlayer.CountStations | ( | RadioFilter | filter = null | ) |
Count all RadioStation for a given RadioFilter.
- Parameters
-
filter Filter for the radio stations (default: null, optional)
- Returns
- Number of all RadioStation for a given RadioFilter.
Implements Crosstales.Radio.Set.ISet.
◆ GetStations()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.SimplePlayer.GetStations | ( | bool | random = false , |
RadioFilter | filter = null |
||
) |
Get all RadioStation for a given RadioFilter.
- Parameters
-
random Return random RadioStation (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All RadioStation for a given RadioFilter.
Implements Crosstales.Radio.Set.ISet.
◆ Load()
void Crosstales.Radio.SimplePlayer.Load | ( | ) |
Loads all stations from this set (via providers).
Implements Crosstales.Radio.Set.ISet.
◆ Mute()
|
virtual |
Mute the playback of the record.
Implements Crosstales.Radio.BasePlayer.
◆ Next() [1/2]
void Crosstales.Radio.SimplePlayer.Next | ( | ) |
Plays the next radio station.
◆ Next() [2/2]
RadioStation Crosstales.Radio.SimplePlayer.Next | ( | bool | random, |
RadioFilter | _filter = null |
||
) |
Plays the next (normal/random) radio station.
- Parameters
-
random Play a random radio station _filter Filter (default: null, optional)
- Returns
- Next RadioStation
◆ NextStation()
RadioStation Crosstales.Radio.SimplePlayer.NextStation | ( | bool | random = false , |
RadioFilter | filter = null |
||
) |
Next (normal/random) radio station from this set.
- Parameters
-
random Return a random radio station (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- Next radio station.
Implements Crosstales.Radio.Set.ISet.
◆ Play()
|
virtual |
Plays the radio-station.
Implements Crosstales.Radio.BasePlayer.
◆ Previous() [1/2]
void Crosstales.Radio.SimplePlayer.Previous | ( | ) |
Plays the previous radio station.
◆ Previous() [2/2]
RadioStation Crosstales.Radio.SimplePlayer.Previous | ( | bool | random, |
RadioFilter | _filter = null |
||
) |
Plays the previous radio station.
- Parameters
-
random Play a random radio station _filter Filter (default: null, optional)
- Returns
- Previous RadioStation
◆ PreviousStation()
RadioStation Crosstales.Radio.SimplePlayer.PreviousStation | ( | bool | random = false , |
RadioFilter | filter = null |
||
) |
Previous (normal/random) radio station from this set.
- Parameters
-
random Return a random radio station (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- Previous radio station.
Implements Crosstales.Radio.Set.ISet.
◆ RandomizeStations()
void Crosstales.Radio.SimplePlayer.RandomizeStations | ( | bool | resetIndex = true | ) |
Randomize all radio stations.
- Parameters
-
resetIndex Reset the index of the random radio stations (default: true, optional)
Implements Crosstales.Radio.Set.ISet.
◆ Restart()
|
virtual |
Restarts the playback of the radio-station.
- Parameters
-
invokeDelay Delay for the restart (default: 0.4, optional)
Implements Crosstales.Radio.BasePlayer.
◆ Save()
void Crosstales.Radio.SimplePlayer.Save | ( | string | path, |
RadioFilter | filter = null |
||
) |
Saves all stations from this set as text-file with streams.
- Parameters
-
path Path to the text-file. filter Filter for the radio stations (default: null, optional)
Implements Crosstales.Radio.Set.ISet.
◆ StationFromHashCode()
RadioStation Crosstales.Radio.SimplePlayer.StationFromHashCode | ( | int | hashCode | ) |
Radio station from a hashcode from this set.
- Parameters
-
hashCode Hashcode of the radio station
- Returns
- Radio station from hashcode.
Implements Crosstales.Radio.Set.ISet.
◆ StationFromIndex()
RadioStation Crosstales.Radio.SimplePlayer.StationFromIndex | ( | bool | random = false , |
int | index = -1 , |
||
RadioFilter | filter = null |
||
) |
Radio station from a given index (normal/random) from this set.
- Parameters
-
random Return a random Radio station (default: false, optional) index Index of the radio station (default: -1, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- Record from index.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByBitrate()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.SimplePlayer.StationsByBitrate | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by bitrate.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by bitrate.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByCities()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.SimplePlayer.StationsByCities | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by cities.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by cities.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByCountries()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.SimplePlayer.StationsByCountries | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by countries.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by countries.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByFormat()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.SimplePlayer.StationsByFormat | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by audio format.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by audio format.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByGenres()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.SimplePlayer.StationsByGenres | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by genres.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by genre.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByLanguages()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.SimplePlayer.StationsByLanguages | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by languages.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by languages.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByName()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.SimplePlayer.StationsByName | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by name.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by name.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByRating()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.SimplePlayer.StationsByRating | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by rating.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by rating.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByStation()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.SimplePlayer.StationsByStation | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by station.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by station.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByURL()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.SimplePlayer.StationsByURL | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by URL.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by URL.
Implements Crosstales.Radio.Set.ISet.
◆ Stop()
|
virtual |
Stops the playback of the radio-station.
Implements Crosstales.Radio.BasePlayer.
◆ UnMute()
|
virtual |
Unmute the playback of the record.
Implements Crosstales.Radio.BasePlayer.
Property Documentation
◆ Filter
|
getset |
Global RadioFilter (active if no explicit filter is given).
◆ FollowDirection
|
getset |
In case 'Next' or 'Previous' is called, follow the logical direction through the playlist.
◆ PlayEndless
|
getset |
Enable endless play.
◆ Player
|
getset |
'RadioPlayer' from the scene.
◆ PlayOnStart
|
getset |
Play a radio on start.
◆ PlayRandom
|
getset |
Play the radio stations in random order.
◆ Retries
|
getset |
Defines how many times should the radio station restart after an error before giving up.
◆ RetryOnError
|
getset |
Retry to start the radio on an error.
◆ Set
|
getset |
'RadioSet' from the scene.
Event Documentation
◆ OnFilterChange
FilterChange Crosstales.Radio.SimplePlayer.OnFilterChange |
An event triggered whenever the filter changes.
◆ OnProviderReady
ProviderReady Crosstales.Radio.SimplePlayer.OnProviderReady |
An event triggered whenever all providers are ready.
◆ OnStationChange
StationChange Crosstales.Radio.SimplePlayer.OnStationChange |
An event triggered whenever an radio station changes.
◆ OnStationsChange
StationsChange Crosstales.Radio.SimplePlayer.OnStationsChange |
An event triggered whenever the stations change.
The documentation for this class was generated from the following file:
- C:/Users/slaub/Unity/assets/Radio/RadioPro/Assets/Plugins/crosstales/Radio/Scripts/SimplePlayer.cs