Radio manager for multiple radio players. More...
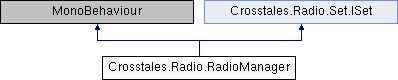
Public Member Functions | |
System.Collections.Generic.List< RadioPlayer > | GetPlayers (bool random=false, RadioFilter filter=null) |
Get all RadioPlayer for a given RadioFilter. More... | |
int | CountPlayers (RadioFilter filter=null) |
Count all RadioPlayer for a given RadioFilter. More... | |
void | PlayAll () |
Play all radios of this manager at once. More... | |
RadioPlayer | PlayerFromIndex (bool random=false, int index=-1, RadioFilter filter=null) |
Radio player from a given index (normal/random) from this manager. More... | |
RadioPlayer | Next (bool random=false, RadioFilter filter=null, bool stopAll=true, bool playImmediately=true) |
Next (normal/random) radio from this manager. More... | |
RadioPlayer | Previous (bool random=false, RadioFilter filter=null, bool stopAll=true, bool playImmediately=true) |
Previous (normal/random) radio from this manager. More... | |
void | StopAll (bool resetIndex) |
Stops all radios of this manager at once. More... | |
void | StopAll () |
Stops all radios of this manager at once. More... | |
System.Collections.Generic.List< RadioPlayer > | PlayersByName (bool desc=false, RadioFilter filter=null) |
Returns all radios of this manager ordered by name. More... | |
System.Collections.Generic.List< RadioPlayer > | PlayersByURL (bool desc=false, RadioFilter filter=null) |
Returns all radios of this manager ordered by URL. More... | |
System.Collections.Generic.List< RadioPlayer > | PlayersByFormat (bool desc=false, RadioFilter filter=null) |
Returns all radios of this manager ordered by audio format. More... | |
System.Collections.Generic.List< RadioPlayer > | PlayersByStation (bool desc=false, RadioFilter filter=null) |
Returns all radios of this manager ordered by station. More... | |
System.Collections.Generic.List< RadioPlayer > | PlayersByBitrate (bool desc=false, RadioFilter filter=null) |
Returns all radios of this manager ordered by bitrate. More... | |
System.Collections.Generic.List< RadioPlayer > | PlayersByGenres (bool desc=false, RadioFilter filter=null) |
Returns all radios of this manager ordered by genres. More... | |
System.Collections.Generic.List< RadioPlayer > | PlayersByRating (bool desc=false, RadioFilter filter=null) |
Returns all radios of this manager ordered by rating. More... | |
void | RandomizePlayers (bool resetIndex=true) |
Randomize all radio players. More... | |
override string | ToString () |
System.Collections.Generic.List< RadioStation > | GetStations (bool random=false, RadioFilter filter=null) |
Get all RadioStation for a given RadioFilter. More... | |
int | CountStations (RadioFilter filter=null) |
Count all RadioStation for a given RadioFilter. More... | |
RadioStation | StationFromIndex (bool random=false, int index=-1, RadioFilter filter=null) |
Radio station from a given index (normal/random) from this set. More... | |
RadioStation | StationFromHashCode (int hashCode) |
Radio station from a hashcode from this set. More... | |
RadioStation | NextStation (bool random=false, RadioFilter filter=null) |
Next (normal/random) radio station from this set. More... | |
RadioStation | PreviousStation (bool random=false, RadioFilter filter=null) |
Previous (normal/random) radio station from this set. More... | |
System.Collections.Generic.List< RadioStation > | StationsByName (bool desc=false, RadioFilter filter=null) |
Returns all radio stations of this set ordered by name. More... | |
System.Collections.Generic.List< RadioStation > | StationsByURL (bool desc=false, RadioFilter filter=null) |
Returns all radio stations of this set ordered by URL. More... | |
System.Collections.Generic.List< RadioStation > | StationsByFormat (bool desc=false, RadioFilter filter=null) |
Returns all radio stations of this set ordered by audio format. More... | |
System.Collections.Generic.List< RadioStation > | StationsByStation (bool desc=false, RadioFilter filter=null) |
Returns all radio stations of this set ordered by station. More... | |
System.Collections.Generic.List< RadioStation > | StationsByBitrate (bool desc=false, RadioFilter filter=null) |
Returns all radio stations of this set ordered by bitrate. More... | |
System.Collections.Generic.List< RadioStation > | StationsByGenres (bool desc=false, RadioFilter filter=null) |
Returns all radio stations of this set ordered by genres. More... | |
System.Collections.Generic.List< RadioStation > | StationsByCities (bool desc=false, RadioFilter filter=null) |
Returns all radio stations of this set ordered by cities. More... | |
System.Collections.Generic.List< RadioStation > | StationsByCountries (bool desc=false, RadioFilter filter=null) |
Returns all radio stations of this set ordered by countries. More... | |
System.Collections.Generic.List< RadioStation > | StationsByLanguages (bool desc=false, RadioFilter filter=null) |
Returns all radio stations of this set ordered by languages. More... | |
System.Collections.Generic.List< RadioStation > | StationsByRating (bool desc=false, RadioFilter filter=null) |
Returns all radio stations of this set ordered by rating. More... | |
void | Load () |
Loads all stations from this set (via providers). More... | |
void | Save (string path, RadioFilter filter=null) |
Saves all stations from this set as text-file with streams. More... | |
void | RandomizeStations (bool resetIndex=true) |
Randomize all radio stations. More... | |
Public Attributes | |
RadioSet | Set |
'Set' from the scene. More... | |
RadioFilter | Filter |
Global RadioFilter (active if no explicit filter is given). More... | |
bool | LoadOnStart |
Calls 'Load' on Start (default: false). More... | |
bool | LoadOnStartInEditor |
Calls 'Load' on Start in Editor (default: false). More... | |
bool | InstantiateRadioPlayers |
Instantiate RadioPlayer (default: false). More... | |
GameObject | RadioPrefab |
Prefab of the RadioPlayer. More... | |
System.Collections.Generic.List< RadioPlayer > | Players => players |
List of all instantiated RadioPlayer. More... | |
FilterChangeEvent | OnFilterChanged |
StationsChangeEvent | OnStationsChanged |
ProviderReadyEvent | OnProviderReadyEvent |
System.Collections.Generic.List< RadioStation > | Stations => Set != null ? Set.Stations : new System.Collections.Generic.List<RadioStation>() |
System.Collections.Generic.List< RadioStation > | RandomStations => Set != null ? Set.RandomStations : new System.Collections.Generic.List<RadioStation>() |
bool | isReady => Set != null && Set.isReady |
Properties | |
bool | isPlayback [get] |
Is any of the RadioPlayers in playback-mode? More... | |
bool | isAudioPlaying [get] |
Is any of the RadioPlayers playing audio? More... | |
bool | isBuffering [get] |
Is any of the RadioPlayers buffering? More... | |
int? | CurrentStationIndex [get, set] |
int? | CurrentRandomStationIndex [get, set] |
![]() | |
System.Collections.Generic.List< RadioStation > | Stations [get] |
List of all loaded RadioStation from all providers. More... | |
System.Collections.Generic.List< RadioStation > | RandomStations [get] |
Returns the list of all randomized RadioStation from this set. More... | |
bool | isReady [get] |
Are all providers of this set ready (= data loaded)? More... | |
int | CurrentStationIndex [get, set] |
Current station index. More... | |
int | CurrentRandomStationIndex [get, set] |
Current random station index. More... | |
Events | |
FilterChange | OnFilterChange |
An event triggered whenever the filter changes. More... | |
StationsChange | OnStationsChange |
An event triggered whenever the stations change. More... | |
ProviderReady | OnProviderReady |
An event triggered whenever all providers are ready. More... | |
Detailed Description
Radio manager for multiple radio players.
Member Function Documentation
◆ CountPlayers()
int Crosstales.Radio.RadioManager.CountPlayers | ( | RadioFilter | filter = null | ) |
Count all RadioPlayer for a given RadioFilter.
- Parameters
-
filter Filter for the radio players (default: null, optional)
- Returns
- Number of all RadioPlayer for a given RadioFilter.
◆ CountStations()
int Crosstales.Radio.RadioManager.CountStations | ( | RadioFilter | filter = null | ) |
Count all RadioStation for a given RadioFilter.
- Parameters
-
filter Filter for the radio stations (default: null, optional)
- Returns
- Number of all RadioStation for a given RadioFilter.
Implements Crosstales.Radio.Set.ISet.
◆ GetPlayers()
System.Collections.Generic.List<RadioPlayer> Crosstales.Radio.RadioManager.GetPlayers | ( | bool | random = false , |
RadioFilter | filter = null |
||
) |
Get all RadioPlayer for a given RadioFilter.
- Parameters
-
random Return random RadioPlayer (default: false, optional) filter Filter for the radio players (default: null, optional)
- Returns
- All RadioPlayer for a given RadioFilter.
◆ GetStations()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.RadioManager.GetStations | ( | bool | random = false , |
RadioFilter | filter = null |
||
) |
Get all RadioStation for a given RadioFilter.
- Parameters
-
random Return random RadioStation (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All RadioStation for a given RadioFilter.
Implements Crosstales.Radio.Set.ISet.
◆ Load()
void Crosstales.Radio.RadioManager.Load | ( | ) |
Loads all stations from this set (via providers).
Implements Crosstales.Radio.Set.ISet.
◆ Next()
RadioPlayer Crosstales.Radio.RadioManager.Next | ( | bool | random = false , |
RadioFilter | filter = null , |
||
bool | stopAll = true , |
||
bool | playImmediately = true |
||
) |
Next (normal/random) radio from this manager.
- Parameters
-
random Return a random radio player (default: false, optional) filter Filter for the radio players (default: null, optional) stopAll Stops all radios of this manager (default: true, optional) playImmediately Plays the radio (default: true, optional)
- Returns
- Next radio station.
◆ NextStation()
RadioStation Crosstales.Radio.RadioManager.NextStation | ( | bool | random = false , |
RadioFilter | filter = null |
||
) |
Next (normal/random) radio station from this set.
- Parameters
-
random Return a random radio station (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- Next radio station.
Implements Crosstales.Radio.Set.ISet.
◆ PlayAll()
void Crosstales.Radio.RadioManager.PlayAll | ( | ) |
Play all radios of this manager at once.
◆ PlayerFromIndex()
RadioPlayer Crosstales.Radio.RadioManager.PlayerFromIndex | ( | bool | random = false , |
int | index = -1 , |
||
RadioFilter | filter = null |
||
) |
◆ PlayersByBitrate()
System.Collections.Generic.List<RadioPlayer> Crosstales.Radio.RadioManager.PlayersByBitrate | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radios of this manager ordered by bitrate.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio players (default: null, optional)
- Returns
- All radios of this manager ordered by bitrate.
◆ PlayersByFormat()
System.Collections.Generic.List<RadioPlayer> Crosstales.Radio.RadioManager.PlayersByFormat | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radios of this manager ordered by audio format.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio players (default: null, optional)
- Returns
- All radios of this manager ordered by audio format.
◆ PlayersByGenres()
System.Collections.Generic.List<RadioPlayer> Crosstales.Radio.RadioManager.PlayersByGenres | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radios of this manager ordered by genres.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio players (default: null, optional)
- Returns
- All radios of this manager ordered by genre.
◆ PlayersByName()
System.Collections.Generic.List<RadioPlayer> Crosstales.Radio.RadioManager.PlayersByName | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radios of this manager ordered by name.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio players (default: null, optional)
- Returns
- All radios of this manager ordered by name.
◆ PlayersByRating()
System.Collections.Generic.List<RadioPlayer> Crosstales.Radio.RadioManager.PlayersByRating | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radios of this manager ordered by rating.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio players (default: null, optional)
- Returns
- All radios of this manager ordered by rating.
◆ PlayersByStation()
System.Collections.Generic.List<RadioPlayer> Crosstales.Radio.RadioManager.PlayersByStation | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radios of this manager ordered by station.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio players (default: null, optional)
- Returns
- All radios of this manager ordered by station.
◆ PlayersByURL()
System.Collections.Generic.List<RadioPlayer> Crosstales.Radio.RadioManager.PlayersByURL | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radios of this manager ordered by URL.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio players (default: null, optional)
- Returns
- All radios of this manager ordered by URL.
◆ Previous()
RadioPlayer Crosstales.Radio.RadioManager.Previous | ( | bool | random = false , |
RadioFilter | filter = null , |
||
bool | stopAll = true , |
||
bool | playImmediately = true |
||
) |
Previous (normal/random) radio from this manager.
- Parameters
-
random Return a random radio player (default: false, optional) filter Filter for the radio players (default: null, optional) stopAll Stops all radios of this manager (default: true, optional) playImmediately Plays the radio (default: true, optional)
- Returns
- Previous radio station.
◆ PreviousStation()
RadioStation Crosstales.Radio.RadioManager.PreviousStation | ( | bool | random = false , |
RadioFilter | filter = null |
||
) |
Previous (normal/random) radio station from this set.
- Parameters
-
random Return a random radio station (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- Previous radio station.
Implements Crosstales.Radio.Set.ISet.
◆ RandomizePlayers()
void Crosstales.Radio.RadioManager.RandomizePlayers | ( | bool | resetIndex = true | ) |
Randomize all radio players.
- Parameters
-
resetIndex Reset the index of the random radio stations (default: true, optional)
◆ RandomizeStations()
void Crosstales.Radio.RadioManager.RandomizeStations | ( | bool | resetIndex = true | ) |
Randomize all radio stations.
- Parameters
-
resetIndex Reset the index of the random radio stations (default: true, optional)
Implements Crosstales.Radio.Set.ISet.
◆ Save()
void Crosstales.Radio.RadioManager.Save | ( | string | path, |
RadioFilter | filter = null |
||
) |
Saves all stations from this set as text-file with streams.
- Parameters
-
path Path to the text-file. filter Filter for the radio stations (default: null, optional)
Implements Crosstales.Radio.Set.ISet.
◆ StationFromHashCode()
RadioStation Crosstales.Radio.RadioManager.StationFromHashCode | ( | int | hashCode | ) |
Radio station from a hashcode from this set.
- Parameters
-
hashCode Hashcode of the radio station
- Returns
- Radio station from hashcode.
Implements Crosstales.Radio.Set.ISet.
◆ StationFromIndex()
RadioStation Crosstales.Radio.RadioManager.StationFromIndex | ( | bool | random = false , |
int | index = -1 , |
||
RadioFilter | filter = null |
||
) |
Radio station from a given index (normal/random) from this set.
- Parameters
-
random Return a random Radio station (default: false, optional) index Index of the radio station (default: -1, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- Record from index.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByBitrate()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.RadioManager.StationsByBitrate | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by bitrate.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by bitrate.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByCities()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.RadioManager.StationsByCities | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by cities.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by cities.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByCountries()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.RadioManager.StationsByCountries | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by countries.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by countries.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByFormat()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.RadioManager.StationsByFormat | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by audio format.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by audio format.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByGenres()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.RadioManager.StationsByGenres | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by genres.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by genre.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByLanguages()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.RadioManager.StationsByLanguages | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by languages.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by languages.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByName()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.RadioManager.StationsByName | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by name.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by name.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByRating()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.RadioManager.StationsByRating | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by rating.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by rating.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByStation()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.RadioManager.StationsByStation | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by station.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by station.
Implements Crosstales.Radio.Set.ISet.
◆ StationsByURL()
System.Collections.Generic.List<RadioStation> Crosstales.Radio.RadioManager.StationsByURL | ( | bool | desc = false , |
RadioFilter | filter = null |
||
) |
Returns all radio stations of this set ordered by URL.
- Parameters
-
desc Descending order (default: false, optional) filter Filter for the radio stations (default: null, optional)
- Returns
- All radios of this set ordered by URL.
Implements Crosstales.Radio.Set.ISet.
◆ StopAll() [1/2]
void Crosstales.Radio.RadioManager.StopAll | ( | ) |
Stops all radios of this manager at once.
◆ StopAll() [2/2]
void Crosstales.Radio.RadioManager.StopAll | ( | bool | resetIndex | ) |
Stops all radios of this manager at once.
- Parameters
-
resetIndex Reset the index of the radio stations (default: false)
Member Data Documentation
◆ Filter
RadioFilter Crosstales.Radio.RadioManager.Filter |
Global RadioFilter (active if no explicit filter is given).
◆ InstantiateRadioPlayers
bool Crosstales.Radio.RadioManager.InstantiateRadioPlayers |
Instantiate RadioPlayer (default: false).
◆ LoadOnStart
bool Crosstales.Radio.RadioManager.LoadOnStart |
Calls 'Load' on Start (default: false).
◆ LoadOnStartInEditor
bool Crosstales.Radio.RadioManager.LoadOnStartInEditor |
Calls 'Load' on Start in Editor (default: false).
◆ Players
System.Collections.Generic.List<RadioPlayer> Crosstales.Radio.RadioManager.Players => players |
List of all instantiated RadioPlayer.
◆ RadioPrefab
GameObject Crosstales.Radio.RadioManager.RadioPrefab |
Prefab of the RadioPlayer.
◆ Set
Property Documentation
◆ isAudioPlaying
|
get |
Is any of the RadioPlayers playing audio?
- Returns
- True if any of the RadioPlayers is playing audio.
◆ isBuffering
|
get |
Is any of the RadioPlayers buffering?
- Returns
- True if any of the RadioPlayers is buffering.
◆ isPlayback
|
get |
Is any of the RadioPlayers in playback-mode?
- Returns
- True if any of the RadioPlayers is in playback-mode.
Event Documentation
◆ OnFilterChange
FilterChange Crosstales.Radio.RadioManager.OnFilterChange |
An event triggered whenever the filter changes.
◆ OnProviderReady
ProviderReady Crosstales.Radio.RadioManager.OnProviderReady |
An event triggered whenever all providers are ready.
◆ OnStationsChange
StationsChange Crosstales.Radio.RadioManager.OnStationsChange |
An event triggered whenever the stations change.
The documentation for this class was generated from the following file:
- C:/Users/slaub/Unity/assets/Radio/RadioPro/Assets/Plugins/crosstales/Radio/Scripts/RadioManager.cs