Base for various helper functions. More...
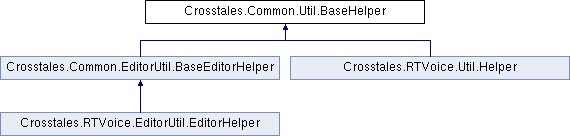
Static Public Member Functions | |
static string | CreateString (string generateChars, int stringLength) |
Creates a string of characters with a given length. More... | |
static System.Collections.Generic.List< string > | SplitStringToLines (string text, bool ignoreCommentedLines=true, int skipHeaderLines=0, int skipFooterLines=0) |
Split the given text to lines and return it as list. More... | |
static string | FormatBytesToHRF (long bytes, bool useSI=false) |
Format byte-value to Human-Readable-Form. More... | |
static string | FormatSecondsToHRF (double seconds) |
Format seconds to Human-Readable-Form. More... | |
static Color | HSVToRGB (float h, float s, float v, float a=1f) |
Generate nice HSV colors. Based on https://gist.github.com/rje/6206099 More... | |
static string | GenerateLoremIpsum (int length, int minSentences=1, int maxSentences=int.MaxValue, int minWords=1, int maxWords=15) |
Generates a "Lorem Ipsum" based on various parameters. More... | |
static string | LanguageToISO639 (SystemLanguage language) |
Converts a SystemLanguage to an ISO639-1 code. Returns "en" if the SystemLanguage could not be converted. More... | |
static SystemLanguage | ISO639ToLanguage (string isoCode) |
Converts an ISO639-1 code to a SystemLanguage. Returns SystemLanguage.English if the code could not be converted. More... | |
static object | InvokeMethod (string className, string methodName, System.Reflection.BindingFlags flags=System.Reflection.BindingFlags.Static|System.Reflection.BindingFlags.Public, params object[] parameters) |
Invokes a method on a full qualified class. More... | |
static string | GetArgument (string name) |
Returns an argument for a name from the url or command line. More... | |
static string[] | GetArguments () |
Returns all arguments from the url or command line. More... | |
static System.Collections.Generic.Dictionary< string, System.Collections.Generic.List< string > > | ParseJSON (string json) |
Parses a given JSON into a dictionary with key and values Note: this is a very basic implementation for simple JSON-strings - don't expect it to work with complex (e.g. nested) JSONs More... | |
Static Public Attributes | |
static bool | ApplicationIsPlaying = Application.isPlaying |
static bool | isEditorMode => isEditor && !ApplicationIsPlaying |
Checks if we are in Editor mode. More... | |
static bool | isStandalonePlatform => isWindowsPlatform || isMacOSPlatform || isLinuxPlatform |
Checks if the current platform is standalone (Windows, macOS or Linux). More... | |
static bool | isWebPlatform => isWebGLPlatform |
Checks if the current platform is Web (WebPlayer or WebGL). More... | |
static bool | isWindowsBasedPlatform => isWindowsPlatform || isWSAPlatform || isXboxOnePlatform |
Checks if the current platform is Windows-based (Windows standalone, WSA or XboxOne). More... | |
static bool | isWSABasedPlatform => isWSAPlatform || isXboxOnePlatform |
Checks if the current platform is WSA-based (WSA or XboxOne). More... | |
static bool | isAppleBasedPlatform => isMacOSPlatform || isIOSPlatform || isTvOSPlatform |
Checks if the current platform is Apple-based (macOS standalone, iOS or tvOS). More... | |
static bool | isIOSBasedPlatform => isIOSPlatform || isTvOSPlatform |
Checks if the current platform is iOS-based (iOS or tvOS). More... | |
static bool | isMobilePlatform => isAndroidPlatform || isIOSBasedPlatform |
Checks if the current platform is mobile (Android and iOS). More... | |
static bool | isEditor => isWindowsEditor || isMacOSEditor || isLinuxEditor |
Checks if we are inside the Editor. More... | |
Static Protected Attributes | |
static readonly System.Random | _rnd = new System.Random() |
Properties | |
static System.Globalization.CultureInfo | BaseCulture [get] |
The current culture of the application. More... | |
static bool | isIL2CPP [get] |
Checks if the current build target uses IL2CPP. More... | |
static Crosstales.Common.Model.Enum.Platform? | CurrentPlatform [get] |
Returns the current platform. More... | |
static int | AndroidAPILevel [get] |
Returns the Android API level of the current device (Android only)". More... | |
static bool | isWindowsPlatform [get] |
Checks if the current platform is Windows. More... | |
static bool | isMacOSPlatform [get] |
Checks if the current platform is OSX. More... | |
static bool | isLinuxPlatform [get] |
Checks if the current platform is Linux. More... | |
static bool | isAndroidPlatform [get] |
Checks if the current platform is Android. More... | |
static bool | isIOSPlatform [get] |
Checks if the current platform is iOS. More... | |
static bool | isTvOSPlatform [get] |
Checks if the current platform is tvOS. More... | |
static bool | isWSAPlatform [get] |
Checks if the current platform is WSA. More... | |
static bool | isXboxOnePlatform [get] |
Checks if the current platform is XboxOne. More... | |
static bool | isPS4Platform [get] |
Checks if the current platform is PS4. More... | |
static bool | isWebGLPlatform [get] |
Checks if the current platform is WebGL. More... | |
static bool | isWindowsEditor [get] |
Checks if we are inside the Windows Editor. More... | |
static bool | isMacOSEditor [get] |
Checks if we are inside the macOS Editor. More... | |
static bool | isLinuxEditor [get] |
Checks if we are inside the Linux Editor. More... | |
Detailed Description
Base for various helper functions.
Member Function Documentation
◆ CreateString()
|
static |
Creates a string of characters with a given length.
- Parameters
-
generateChars Characters to generate the string (if more than one character is used, the generated string will be a randomized result of all characters) stringLength Length of the generated string
- Returns
- Generated string
◆ FormatBytesToHRF()
|
static |
Format byte-value to Human-Readable-Form.
- Parameters
-
bytes Value in bytes useSI Use SI-system (optional, default: false)
- Returns
- Formatted byte-value in Human-Readable-Form.
◆ FormatSecondsToHRF()
|
static |
Format seconds to Human-Readable-Form.
- Parameters
-
seconds Value in seconds
- Returns
- Formatted seconds in Human-Readable-Form.
◆ GenerateLoremIpsum()
|
static |
Generates a "Lorem Ipsum" based on various parameters.
- Parameters
-
length Length of the text minSentences Minimum number of sentences for the text (optional, default: 1) maxSentences Maximal number of sentences for the text (optional, default: int.MaxValue) minWords Minimum number of words per sentence (optional, default: 1) maxWords Maximal number of words per sentence (optional, default: 15)
- Returns
- "Lorem Ipsum" based on the given parameters.
◆ GetArgument()
|
static |
Returns an argument for a name from the url or command line.
- Parameters
-
name Name for the argument
- Returns
- Argument for a name from the url or command line.
◆ GetArguments()
|
static |
Returns all arguments from the url or command line.
- Returns
- Arguments from the url or command line.
◆ HSVToRGB()
|
static |
Generate nice HSV colors. Based on https://gist.github.com/rje/6206099
- Parameters
-
h Hue s Saturation v Value a Alpha (optional)
- Returns
- True if the current platform is supported.
◆ InvokeMethod()
|
static |
Invokes a method on a full qualified class.
- Parameters
-
className Full qualified name of the class methodName Public static method of the class to execute flags Binding flags for the method (optional, default: static/public) parameters Parameters for the method (optional)
◆ ISO639ToLanguage()
|
static |
Converts an ISO639-1 code to a SystemLanguage. Returns SystemLanguage.English if the code could not be converted.
- Parameters
-
isoCode ISO639-1 code to convert.
- Returns
- "SystemLanguage for the given ISO639-1 code.
◆ LanguageToISO639()
|
static |
Converts a SystemLanguage to an ISO639-1 code. Returns "en" if the SystemLanguage could not be converted.
- Parameters
-
language SystemLanguage to convert.
- Returns
- "ISO639-1 code for the given SystemLanguage.
◆ ParseJSON()
|
static |
Parses a given JSON into a dictionary with key and values Note: this is a very basic implementation for simple JSON-strings - don't expect it to work with complex (e.g. nested) JSONs
- Parameters
-
json JSON-string to parse
- Returns
- Dictionary with key and values from the JSON-string
◆ SplitStringToLines()
|
static |
Split the given text to lines and return it as list.
- Parameters
-
text Complete text fragment ignoreCommentedLines Ignore commente lines (optional, default: true) skipHeaderLines Number of skipped header lines (optional, default: 0) skipFooterLines Number of skipped footer lines (optional, default: 0)
- Returns
- Splitted lines as array
Member Data Documentation
◆ isAppleBasedPlatform
|
static |
Checks if the current platform is Apple-based (macOS standalone, iOS or tvOS).
- Returns
- True if the current platform is Apple-based (macOS standalone, iOS or tvOS).
◆ isEditor
|
static |
Checks if we are inside the Editor.
- Returns
- True if we are inside the Editor.
◆ isEditorMode
|
static |
Checks if we are in Editor mode.
- Returns
- True if in Editor mode.
◆ isIOSBasedPlatform
|
static |
Checks if the current platform is iOS-based (iOS or tvOS).
- Returns
- True if the current platform is iOS-based (iOS or tvOS).
◆ isMobilePlatform
|
static |
Checks if the current platform is mobile (Android and iOS).
- Returns
- True if the current platform is mobile (Android and iOS).
◆ isStandalonePlatform
|
static |
Checks if the current platform is standalone (Windows, macOS or Linux).
- Returns
- True if the current platform is standalone (Windows, macOS or Linux).
◆ isWebPlatform
|
static |
Checks if the current platform is Web (WebPlayer or WebGL).
- Returns
- True if the current platform is Web (WebPlayer or WebGL).
◆ isWindowsBasedPlatform
|
static |
Checks if the current platform is Windows-based (Windows standalone, WSA or XboxOne).
- Returns
- True if the current platform is Windows-based (Windows standalone, WSA or XboxOne).
◆ isWSABasedPlatform
|
static |
Checks if the current platform is WSA-based (WSA or XboxOne).
- Returns
- True if the current platform is WSA-based (WSA or XboxOne).
Property Documentation
◆ AndroidAPILevel
|
staticget |
Returns the Android API level of the current device (Android only)".
- Returns
- The Android API level of the current device.
◆ BaseCulture
|
staticget |
The current culture of the application.
- Returns
- Culture of the application.
◆ CurrentPlatform
|
staticget |
Returns the current platform.
- Returns
- The current platform.
◆ isAndroidPlatform
|
staticget |
Checks if the current platform is Android.
- Returns
- True if the current platform is Android.
◆ isIL2CPP
|
staticget |
Checks if the current build target uses IL2CPP.
- Returns
- True if the current build target uses IL2CPP.
◆ isIOSPlatform
|
staticget |
Checks if the current platform is iOS.
- Returns
- True if the current platform is iOS.
◆ isLinuxEditor
|
staticget |
Checks if we are inside the Linux Editor.
- Returns
- True if we are inside the Linux Editor.
◆ isLinuxPlatform
|
staticget |
Checks if the current platform is Linux.
- Returns
- True if the current platform is Linux.
◆ isMacOSEditor
|
staticget |
Checks if we are inside the macOS Editor.
- Returns
- True if we are inside the macOS Editor.
◆ isMacOSPlatform
|
staticget |
Checks if the current platform is OSX.
- Returns
- True if the current platform is OSX.
◆ isPS4Platform
|
staticget |
Checks if the current platform is PS4.
- Returns
- True if the current platform is PS4.
◆ isTvOSPlatform
|
staticget |
Checks if the current platform is tvOS.
- Returns
- True if the current platform is tvOS.
◆ isWebGLPlatform
|
staticget |
Checks if the current platform is WebGL.
- Returns
- True if the current platform is WebGL.
◆ isWindowsEditor
|
staticget |
Checks if we are inside the Windows Editor.
- Returns
- True if we are inside the Windows Editor.
◆ isWindowsPlatform
|
staticget |
Checks if the current platform is Windows.
- Returns
- True if the current platform is Windows.
◆ isWSAPlatform
|
staticget |
Checks if the current platform is WSA.
- Returns
- True if the current platform is WSA.
◆ isXboxOnePlatform
|
staticget |
Checks if the current platform is XboxOne.
- Returns
- True if the current platform is XboxOne.
The documentation for this class was generated from the following file:
- C:/Users/slaub/Unity/assets/RTVoice/RTVoicePro/Assets/Plugins/crosstales/Common/Scripts/Util/BaseHelper.cs