Checks the Internet availability. More...
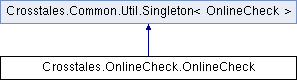
Public Member Functions | |
delegate void | OnlineStatusChange (bool isConnected) |
Callback to determine whether the online status has changed or not. More... | |
delegate void | NetworkReachabilityChange (NetworkReachability networkReachability) |
Callback to determine whether the network reachability has changed or not. More... | |
delegate void | OnlineCheckComplete (bool isConnected, NetworkReachability networkReachability) |
Callback to determine whether the checks have completed or not. More... | |
void | Refresh (bool triggerCallbacks=false) |
Checks for Internet availability. More... | |
IEnumerator | RefreshYield (bool triggerCallbacks=false) |
Checks for Internet availability as an IEnumerator. More... | |
Static Public Member Functions | |
static void | ResetObject () |
Resets this object. More... | |
![]() | |
static void | CreateInstance (bool searchExistingGameObject=true, bool deleteExistingInstance=false) |
Creates an instance of this object. More... | |
static void | DeleteInstance () |
Deletes the instance of this object. More... | |
Public Attributes | |
NetworkReachability | NetworkReachability => networkReachability |
Returns the network reachability. More... | |
bool | isBusy => isRunning |
Returns true if OnlineCheck is busy. More... | |
float | LastCheckRTT => LastCheckRTTMilliseconds / 1000f |
Returns the round trip time of the last successful availability check in seconds. Note: This value is only accurate if used with "ForceWWW" disabled. More... | |
StatusChangeEvent | OnStatusChange |
Protected Member Functions | |
override void | Awake () |
override void | OnApplicationQuit () |
![]() | |
virtual void | OnDestroy () |
Properties | |
bool | EndlessMode [get, set] |
Continuously check for Internet availability within given intervals. More... | |
int? | IntervalMin [get, set] |
Minimum delay between checks in seconds (range: 3 - 59). More... | |
int? | IntervalMax [get, set] |
Maximum delay between checks in seconds (range: 4 - 60). More... | |
int? | Timeout [get, set] |
Timeout for every check in seconds (range: 1 - 10). More... | |
bool | ForceWWW [get, set] |
Force UnityWebRequest instead of WebClient. More... | |
CustomCheck | CustomCheck [get, set] |
Use a custom configuration for the checks. More... | |
bool | Google204 [get, set] |
Enable or disable the 'Google 204' check (279 Bytes). More... | |
bool | GoogleBlank [get, set] |
Enable or disable the 'Google Blank' check (831 Bytes). More... | |
bool | Microsoft [get, set] |
Enable or disable the 'Microsoft' check (184 Bytes). More... | |
bool | Apple [get, set] |
Enable or disable the 'Apple' check (??? Bytes). More... | |
bool | Ubuntu [get, set] |
Enable or disable the 'Ubuntu' check (1001 Bytes). More... | |
bool | RunOnStart [get, set] |
Start at runtime. More... | |
float | Delay [get, set] |
Delay in seconds until the OnlineCheck starts checking. More... | |
bool | isInternetAvailable [get] |
Returns true if an Internet connection is available. More... | |
string | NetworkReachabilityShort [get] |
Returns the network reachability in shorter form. More... | |
System.DateTime | LastCheck [get] |
Returns the time of the last availability check. More... | |
long | DataDownloaded [get] |
Returns the total download size in bytes for the current session. More... | |
int | LastCheckRTTMilliseconds [get] |
Returns the round trip time of the last successful availability check in milliseconds. Note: This value is only accurate if used with "ForceWWW" disabled. More... | |
![]() | |
static T | Instance [get, protected set] |
Returns the singleton instance of this class. More... | |
bool | DontDestroy [get, set] |
Don't destroy gameobject during scene switches. More... | |
Events | |
OnlineStatusChange | OnOnlineStatusChange |
An event triggered whenever the Internet connection status changes. More... | |
NetworkReachabilityChange | OnNetworkReachabilityChange |
An event triggered whenever the network reachability changes. More... | |
OnlineCheckComplete | OnOnlineCheckComplete |
An event triggered whenever the Internet connection check is completed. More... | |
Additional Inherited Members | |
![]() | |
static string | PrefabPath |
Fully qualified prefab path. More... | |
static string | GameObjectName |
Name of the gameobject in the scene. More... | |
![]() | |
static T | instance |
Detailed Description
Checks the Internet availability.
Member Function Documentation
◆ NetworkReachabilityChange()
delegate void Crosstales.OnlineCheck.OnlineCheck.NetworkReachabilityChange | ( | NetworkReachability | networkReachability | ) |
Callback to determine whether the network reachability has changed or not.
◆ OnlineCheckComplete()
delegate void Crosstales.OnlineCheck.OnlineCheck.OnlineCheckComplete | ( | bool | isConnected, |
NetworkReachability | networkReachability | ||
) |
Callback to determine whether the checks have completed or not.
◆ OnlineStatusChange()
delegate void Crosstales.OnlineCheck.OnlineCheck.OnlineStatusChange | ( | bool | isConnected | ) |
Callback to determine whether the online status has changed or not.
◆ Refresh()
void Crosstales.OnlineCheck.OnlineCheck.Refresh | ( | bool | triggerCallbacks = false | ) |
Checks for Internet availability.
- Parameters
-
triggerCallbacks Always trigger the callbacks (default: false, optional)
◆ RefreshYield()
IEnumerator Crosstales.OnlineCheck.OnlineCheck.RefreshYield | ( | bool | triggerCallbacks = false | ) |
Checks for Internet availability as an IEnumerator.
- Parameters
-
triggerCallbacks Always trigger the callbacks (default: false, optional)
◆ ResetObject()
|
static |
Resets this object.
Member Data Documentation
◆ isBusy
bool Crosstales.OnlineCheck.OnlineCheck.isBusy => isRunning |
Returns true if OnlineCheck is busy.
- Returns
- True if if OnlineCheck is busy.
◆ LastCheckRTT
float Crosstales.OnlineCheck.OnlineCheck.LastCheckRTT => LastCheckRTTMilliseconds / 1000f |
Returns the round trip time of the last successful availability check in seconds. Note: This value is only accurate if used with "ForceWWW" disabled.
- Returns
- Round trip time of the last successful availability check in seconds.
◆ NetworkReachability
NetworkReachability Crosstales.OnlineCheck.OnlineCheck.NetworkReachability => networkReachability |
Returns the network reachability.
- Returns
- The Internet reachability.
Property Documentation
◆ Apple
|
getset |
Enable or disable the 'Apple' check (??? Bytes).
◆ CustomCheck
|
getset |
Use a custom configuration for the checks.
◆ DataDownloaded
|
get |
Returns the total download size in bytes for the current session.
- Returns
- Download size in bytes.
◆ Delay
|
getset |
Delay in seconds until the OnlineCheck starts checking.
◆ EndlessMode
|
getset |
Continuously check for Internet availability within given intervals.
◆ ForceWWW
|
getset |
Force UnityWebRequest instead of WebClient.
◆ Google204
|
getset |
Enable or disable the 'Google 204' check (279 Bytes).
◆ GoogleBlank
|
getset |
Enable or disable the 'Google Blank' check (831 Bytes).
◆ IntervalMax
|
getset |
Maximum delay between checks in seconds (range: 4 - 60).
◆ IntervalMin
|
getset |
Minimum delay between checks in seconds (range: 3 - 59).
◆ isInternetAvailable
|
get |
Returns true if an Internet connection is available.
- Returns
- True if an Internet connection is available.
◆ LastCheck
|
get |
Returns the time of the last availability check.
- Returns
- Time of the last availability check.
◆ LastCheckRTTMilliseconds
|
get |
Returns the round trip time of the last successful availability check in milliseconds. Note: This value is only accurate if used with "ForceWWW" disabled.
- Returns
- Round trip time of the last successful availability check in milliseconds.
◆ Microsoft
|
getset |
Enable or disable the 'Microsoft' check (184 Bytes).
◆ NetworkReachabilityShort
|
get |
Returns the network reachability in shorter form.
- Returns
- The Internet reachability in shorter form.
◆ RunOnStart
|
getset |
Start at runtime.
◆ Timeout
|
getset |
Timeout for every check in seconds (range: 1 - 10).
◆ Ubuntu
|
getset |
Enable or disable the 'Ubuntu' check (1001 Bytes).
Event Documentation
◆ OnNetworkReachabilityChange
NetworkReachabilityChange Crosstales.OnlineCheck.OnlineCheck.OnNetworkReachabilityChange |
An event triggered whenever the network reachability changes.
◆ OnOnlineCheckComplete
OnlineCheckComplete Crosstales.OnlineCheck.OnlineCheck.OnOnlineCheckComplete |
An event triggered whenever the Internet connection check is completed.
◆ OnOnlineStatusChange
OnlineStatusChange Crosstales.OnlineCheck.OnlineCheck.OnOnlineStatusChange |
An event triggered whenever the Internet connection status changes.
The documentation for this class was generated from the following file:
- C:/Users/slaub/Unity/assets/OnlineCheck/OnlineCheckPro/Assets/Plugins/crosstales/OnlineCheck/Scripts/OnlineCheck.cs