.NET 8 version of Boga, a collection of tools to make C# development a joyride. 
API:
https://www.crosstales.com/media/data/BogaNet/api/
Packages overview
- BogaNet.Common: Main library for all BogaNet-packages filled with useful helpers to speed up C# development, like helpers for file operations, JSON&XML, strings etc. It also contains many nice extensions, obfuscation and a short UID implementation.
- BogaNet.Avalonia: Little helpers for Avalonia development, like helpers for images and resources.
- BogaNet.Avalonia.Browser: Browser-specific helpers for Avalonia development, like local preferences, i18n, TTS, close/reload callback.
- BogaNet.CRC: Various helpers for cyclic redundancy checks (CRC), namely CRC8, CRC16, CRC32 and CRC64.
- BogaNet.Encoder: A collection of various binary encoders, namely base2, base16, base32, base64, base85 and base91.
- BogaNet.i18n: Localizer for C# applications with CSV files as translations.
- BogaNet.ObfuscatedType: Various obfuscated types for all value types, strings and objects.
- BogaNet.Prefs: Preferences/settings for C# applications. It supports all values types, strings, DateTime and object.
- BogaNet.SecureType: Various encrypted types for all value types, strings and objects.
- BogaNet.TrueRandom: Generates true random numbers from atmospheric noise.
- BogaNet.TTS: Use the built-in Text-To-Speech (TTS) engine under Windows, OSX and Linux.
- BogaNet.Unit: Various units, like area, bit, byte, length, temperature, volume and weight with easy conversion between different types.
BogaNet.Common
Main library for all BogaNet-packages filled with useful helpers to speed up C# development.
Main classes
- FileHelper: Various helper functions for filesystem operations.
- JsonHelper: Helper for JSON operations.
- NetworkHelper: Various helper functions for networking.
- Obfuscator: Obfuscator for strings and byte-arrays.
- ProcessRunner: Executes applications and commands.
- ShortUID: Short Guid implementation with a length of 22 characters (instead 36 of the normal Guid).
- StringHelper: Helper methods for strings.
- XmlHelper: Helper for XML operations.
There are also many powerful extensions for arrays, bytes, dictionaries, lists, numbers and strings.
Nuget:
BogaNet.Common
BogaNet.Avalonia
Little helpers for Avalonia development.
Main classes
i18n
To load translations in Avalonia, move them to the "Assets"-folder. Then use them like this:
ResourceHelper.ResourceAssembly = "YourApp";
Localizer.Instance.LoadResources("Assets/Translation.csv", "Assets/Translation_de.csv");
Localizer.Instance.Culture = new CultureInfo("en");
Console.WriteLine(Localizer.Instance.GetText("Greeting"));
BadWordFilter
To load BWF sources in Avalonia, move them to the "Assets/Filters"-folder. Then use them like this:
ResourceHelper.ResourceAssembly = "YourApp";
BadWordFilter.Instance.LoadResources(true, BWFAvaloniaConstants.BWF_AV_EN, BWFAvaloniaConstants.BWF_AV_DE);
DomainFilter.Instance.LoadResources(BWFAvaloniaConstants.DOMAINS_AV);
string foulText = "MARTIANS are assholes/arschlöcher!!!!!!!!!! => WATCH: https//mytruthpage.com/weirdowatch/martians123.divx or WRITE an EMAIL: weirdo@gmail.com";
string removedProfanity = Pacifier.Instance.ReplaceAll(foulText);
Console.WriteLine(removedProfanity);
Nuget:
BogaNet.Avalonia
BogaNet.Avalonia.Browser
Browser-specific helpers for Avalonia development. It also contains various JavaScript-helper files. This package most likely works with other C# web-technologies like Blazor and Razor, but its untested outside of Avalonia.
Main classes and usage
JavaScript-files:
Unfortunately, you have to manually copy the desired files to "wwwroot" since I don't know how to include it in the Nuget-package correctly... Tips are welcome! :-)
Exit
This callback prevents close and reload-operations in the browser. Add/modify the following code to in "Program.cs" from the Avalonia Browser-project and handle the case (e.g saving data etc.):
private static async Task Main(string[] args)
{
await JSHost.ImportAsync("boganet_exit", "../boganet_exit.js");
}
[JSExport]
internal static void Exit()
{
Preferences.Instance.Save();
}
Preferences
To use the BogaNet.Prefs, add/modify "Program.cs" from the Avalonia Browser-project:
private static async Task Main(string[] args)
{
await JSHost.ImportAsync("boganet_prefs", "../boganet_prefs.js");
Preferences.Instance.Container = new BrowserPreferencesContainer();
await Preferences.Instance.LoadAsync();
}
TTS
To use the BogaNet.TTS, add/modify "Program.cs" from the Avalonia Browser-project:
private static async Task Main(string[] args)
{
await JSHost.ImportAsync("boganet_tts", "../boganet_tts.js");
Speaker.Instance.CustomVoiceProvider = new BrowserVoiceProvider();
}
URL
To set/get the application URL, add/modify "Program.cs" from the Avalonia Browser-project:
private static async Task Main(string[] args)
{
await JSHost.ImportAsync("boganet_url", "../boganet_url.js");
}
The URL can then be accessed via UrlHelper:
Console.WriteLine("Browser-URL: " + UrlHelper.URL);
Nuget:
BogaNet.Avalonia.Browser
BogaNet.BadWordFilter
The “Bad Word Filter” (aka profanity or obscenity filter) is a fresh implementation for .NET8 of the well-known Unity package BadWordFilter PRO and is exactly what the title suggests: a tool to filter swearwords and other “bad sentences”. The library already includes support for over 5’000 of regular expressions (equivalent to tens of thousands of word variations) in 25 languages: Arabic, Chinese, Czech, Danish, Dutch, English, Finnish, French, German, Greek, Hindi, Hungarian, Italian, Japanese, Korean, Norwegian, Persian, Polish, Portuguese, Russian, Spanish, Swedish, Thai, Turkish and Vietnamese.
Fell free to add any words and languages that are missing!
BWF also includes those additional filters:
- Domains (URLs/emails)
- Global bad words
- Emojis (miscellaneous symbols)
- Excessive capitalization
- Excessive punctuation.
It supports any language and any writing system.
Main classes and example code
BadWordFilter.Instance.LoadFiles(true, BWFConstants.BWF_LTR);
BadWordFilter.Instance.LoadFiles(false, BWFConstants.BWF_RTL);
DomainFilter.Instance.LoadFiles(BWFConstants.DOMAINS);
string foulText = "MARTIANS are assholes/arschlöcher!!!!!!!!!! => WATCH: https//mytruthpage.com/weirdowatch/martians123.divx or WRITE an EMAIL: weirdo@gmail.com";
bool contains = Pacifier.Instance.Contains(foulText);
Console.WriteLine("Contains: " + contains);
var allBaddies = Pacifier.Instance.GetAll(foulText);
Console.WriteLine(allBaddies.BNDump());
string removedProfanity = Pacifier.Instance.ReplaceAll(foulText);
Console.WriteLine(removedProfanity);
Nuget:
BogaNet.BadWordFilter
BogaNet.CRC
Various helpers for cyclic redundancy checks (CRC), namely CRC8, CRC16, CRC32 and CRC64.
Main classes
- CRC8: Implementation of CRC with 8bit (byte).
- CRC16: Implementation of CRC with 16bit (ushort).
- CRC32: Implementation of CRC with 32bit (uint).
- CRC64: Implementation of CRC with 64bit (ulong).
Nuget:
BogaNet.CRC
BogaNet.Crypto
Various helpers for cryptographic functions, like hashing (SHA), asymmetric (AES) and symmetric (RSA) encryption/decryption, and HMAC.
Main classes
- AESHelper: Helper for AES cryptography.
- HashHelper: Helper for hash computations. It contains ready-to-use implementations of SHA256, SHA384, SHA512, SHA3-256, SHA3-384 and SHA3-512.
- HMACHelper: Helper for HMAC cryptography. It contains ready-to-use implementations of HMAC-SHA256, HMAC-SHA384, HMAC-SHA512, HMAC-SHA3-256, HMAC-SHA3-384 and HMAC-SHA3-512.
- RSAHelper: Helper for RSA cryptography and X509 certificates.
Nuget:
BogaNet.Crypto
BogaNet.Encoder
A collection of various binary encoders, namely base2, base16, base32, base64, base85 and base91.
Main classes
Nuget:
BogaNet.Encoder
BogaNet.i18n
Localizer for C# applications with CSV files as translations.
Main class and example code
Localizer.Instance.LoadFiles("./Resources/Translation.csv", "./Resources/Translation_de.csv");
Localizer.Instance.Culture = new CultureInfo("en");
Console.WriteLine(Localizer.Instance.GetText("Greeting"));
The files for the translations are CSV-based with the following structure for the columns:
- Key
- Language code (ISO 639) and translation for the key
- Additional column per language code and translation
key,en,de
Greeting,"Hi there!","Hallöchen zusammen!"
Name,"Name:","Name:"
Name_Tooltip,"Enter your name to access the application.","Gib deinen Namen ein um Zugang zur Applikation zu erhalten."
Name_Placeholder,"Enter your name here...","Gib deinen Namen hier ein..."
It's recommended to use one file per language code.
Nuget:
BogaNet.i18n
BogaNet.ObfuscatedType
Various obfuscated types for all value types, strings and objects. This types prevent the values from being "plain" in memory and offers some protection against bad actors (like memory scanners and searchers).
Important note
This types are fast and lightweight, but not cryptographically secure! Use it for less sensitive data, like:
- Username
- First and last names
- Email addresses
- Mailing addresses
- Phone numbers
- Social media profile names
- Highscores
For sensitive data, like passwords etc., it is strongly recommended to use BogaNet.SecureType instead.
Main classes and example code
Obfuscated types for:
DoubleObf age = 35.8;
double years = 7;
age += years;
Console.WriteLine(age.ToString());
StringObf text = "Hello Wörld!";
string frag = " byebye!";
text += frag;
Console.WriteLine(text);
Nuget:
BogaNet.ObfuscatedType
BogaNet.Prefs
Handles preferences/settings for C# applications. It supports all values types, strings, DateTime and object. Furthermore, it allows to store the data in obfuscated form to prevent it from being easily read/modified. The data is automatically stored at application exit.
Main class and example code
string textObf = "Hello obfuscated wörld!";
string keyObf = "textObf";
Preferences.Instance.Set(keyObf, textObf, true);
Console.WriteLine(Preferences.Instance.GetString(keyObf, true));
double number = 12.345;
string keyNumber = "number";
Preferences.Instance.Set(keyNumber, number);
Console.WriteLine(Preferences.Instance.GetNumber<double>(keyNumber).ToString());
Nuget:
BogaNet.Prefs
BogaNet.SecureType
Various encrypted types for all value types, strings and objects. This types prevent the values from being "plain" in memory and offers high protection against bad actors (like memory scanners and searchers).
Important note
This types are performance and memory intense compared to the original C# types, but are cryptographically secure! Use it for sensitive data, like:
- Passwords
- Bank account/routing numbers
- Social security numbers (SSN)
- Drivers license numbers
- Passport ID
- Federal tax ID
- Employer identification numbers (EIN)
- Health insurance policy/member numbers
For less sensitive data, like usernames etc., consider using BogaNet.ObfuscatedType.
Main classes and example code
Secure types for:
DoubleSec age = 35.8;
double years = 7;
age += years;
Console.WriteLine(age.ToString());
StringSec text = "Hello Wörld!";
string frag = " byebye!";
text += frag;
Console.WriteLine(text);
Nuget:
BogaNet.SecureType
BogaNet.TrueRandom
“TrueRandom” is a new implementation for .NET8 of the battle proofed Unity package TrueRandom PRO.
Why use TrueRandom?
“TrueRandom” can generate random numbers and they are “truly random”, because they are generated with atmospheric noise, which supersedes the pseudo-random number algorithms typically use in computer programs. TrueRandom can be used for holding drawings, lotteries and sweepstakes, to drive online games, for scientific applications and for art and music.
Here some more information regarding “true” vs. “pseudo-” random: There are two principal methods used to generate random numbers. The first method measures some physical phenomenon that is expected to be random and then compensates for possible biases in the measurement process. Example sources include measuring atmospheric noise, thermal noise, and other external electromagnetic and quantum phenomena. For example, cosmic background radiation or radioactive decay as measured over short timescales represent sources of natural entropy. The second method uses computational algorithms that can produce long sequences of apparently random results, which are in fact completely determined by a shorter initial value, known as a seed value or key. As a result, the entire seemingly random sequence can be reproduced if the seed value is known. This type of random number generator is often called a pseudorandom number generator. This type of generator typically does not rely on sources of naturally occurring entropy, though it may be periodically seeded by natural sources. This generator type is non-blocking, so they are not rate-limited by an external event, making large bulk reads a possibility. 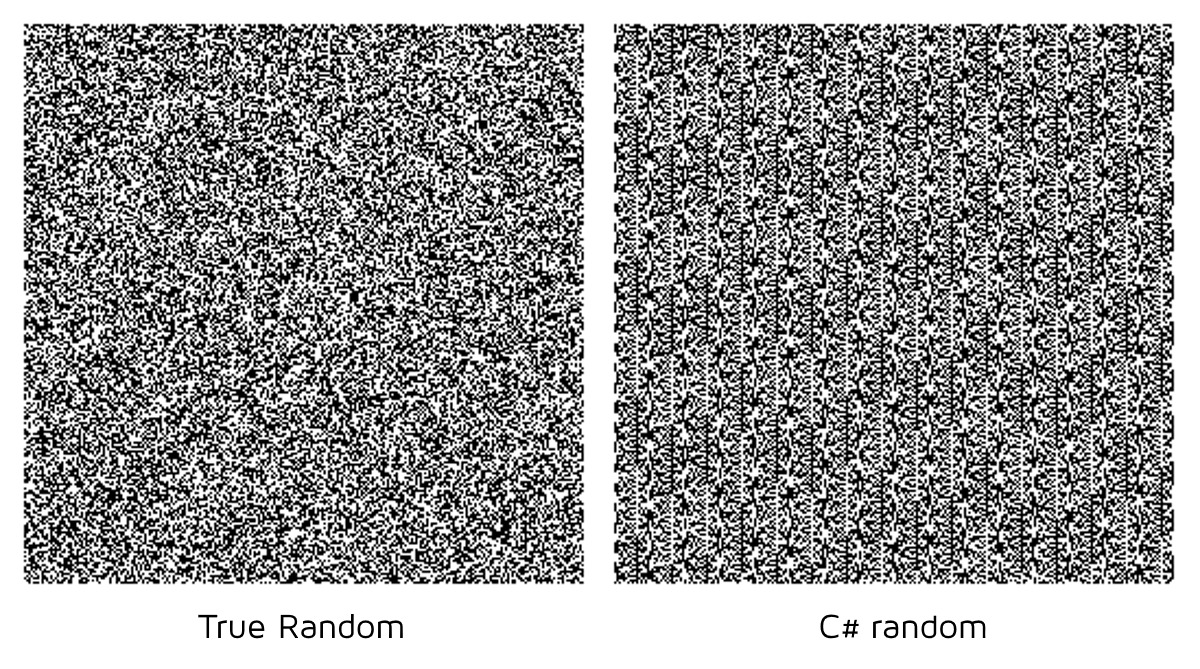
For more, please read this: https://en.wikipedia.org/wiki/Random_number_generation
Quota
"TrueRandom" uses the API of random.org, which provides a free tier with a quota limitation of 1'000'000 random bits per IP-address in 24 hours. This allows to generate at least:
- 120'000 bytes
- 30'000 integers/floats (depends on the size)
- 12'000 strings (length of 10 chars, depends on the settings)
- 3'000 sequences (interval of 10 elements)
If the quota expires, C# pseudo-random will be used automatically. It is recommended to use "TrueRandom" only to set seeds in the PRNG and refresh them as desired to reduce the delay and quota usage.
Main classes
- CheckQuota: Gets the remaining quota from www.random.org.
- BytesTRNG: Generates true random byte-arrays in configurable intervals.
- FloatTRNG: Generates true random floats in configurable intervals.
- IntegerTRNG: Generates true random integers in configurable intervals.
- SequenceTRNG: Randomizes a given interval of integers, i.e. arrange them in random order.
- StringTRNG: Generates true random strings of various length and character compositions.
Nuget:
BogaNet.TrueRandom
BogaNet.TTS
This library is a new implementation for .NET8 of the highly-regarded Unity package RT-Voice PRO and uses the built-in Text-To-Speech (TTS) engine under Windows, OSX and Linux. It provides all installed voices and support for SSML. For an implementation for the web browser, please check my other package BogaNet.Avalonia.Browser. It's also possible to implement your own custom voice provider (e.g. to use other engines like AWS Polly, Azure, Google, ElvenLabs etc.).
Note
The LinuxVoiceProvider uses eSpeak/eSpeak-NG as engine, which is also available for Windows and OSX. Therefore it's possible to take advantage of this engine by installing it and setting the property "UseESpeak" on the Speaker-class to true.
Windows
BogaNet.TTS uses the SAPI-voices, visible by running the following command: windir%\sysWOW64\speech\SpeechUX\SAPI.cpl
Main classes and example code
- Speaker: Main component for TTS-operations.
- Voice: Model for a voice.
Speaker.Instance.Speak("Hello dear user, how are you?");
var voice = Speaker.Instance.VoiceForCulture("de");
Speaker.Instance.SpeakAsync("Hallo lieber Benutzer, wie geht es dir?", voice);
Nuget:
BogaNet.TTS
BogaNet.Unit
Various units, like area, bit, byte, length, temperature, volume and weight with easy conversion between different types.
Units and example code
decimal yard2 = AreaUnit.M2.Convert(AreaUnit.YARD2, 12);
decimal kbit = BitUnit.BIT.Convert(BitUnit.kbit, 1200);
decimal kB = ByteUnit.BYTE.Convert(ByteUnit.kB, 1976);
decimal meter = LengthUnit.YARD.Convert(LengthUnit.M, 9);
decimal kelvin = TemperatureUnit.FAHRENHEIT.Convert(TemperatureUnit.KELVIN, 7800);
decimal pint = VolumeUnit.LITER.Convert(VolumeUnit.PINT_US, 5);
decimal pound = WeightUnit.GRAM.Convert(WeightUnit.POUND, 150);
Nuget:
BogaNet.Unit